Introduction
In MERN (MongoDB, Express, React, Node.js) applications, managing state effectively is crucial for smooth data flow between components. Two popular solutions for state management are Redux and Context API. While both serve similar purposes, they differ in complexity, scalability, and use cases. Refer to the MERN Stack Developer Course for more information. Choosing the right one depends on the size and complexity of the application. This article explores the advantages of each to help make an informed decision.
Overview Of Redux Or Context API In MERN Applications
In MERN (MongoDB, Express, React, Node.js) applications, state management is crucial for handling the data flow across different components. Two popular tools for managing state in React are Redux and Context API.
Redux is a powerful library for state management, especially in large-scale applications. It follows a centralized approach where the entire state of the application is stored in a single global store. Actions are dispatched to modify the state, which are then handled by reducers. Redux provides features like middleware (e.g., Redux Thunk) to manage asynchronous operations and improves debugging through devtools. Its strength lies in scalability and maintainability, particularly for complex applications with deep component hierarchies.
On the other hand, Context API is built into React and is simpler to implement for smaller applications. It allows you to share state across components without the need for prop drilling. Using the Context API, you create a context that holds state and provide it to components that need access to it. It is ideal for moderate-sized applications but may become less efficient in large apps due to unnecessary re-renders.
In short, Redux is better suited for complex applications requiring a global state, while Context API is a simpler, more lightweight alternative for smaller projects.
Advantages Of Using Redux Or Context API In MERN Applications
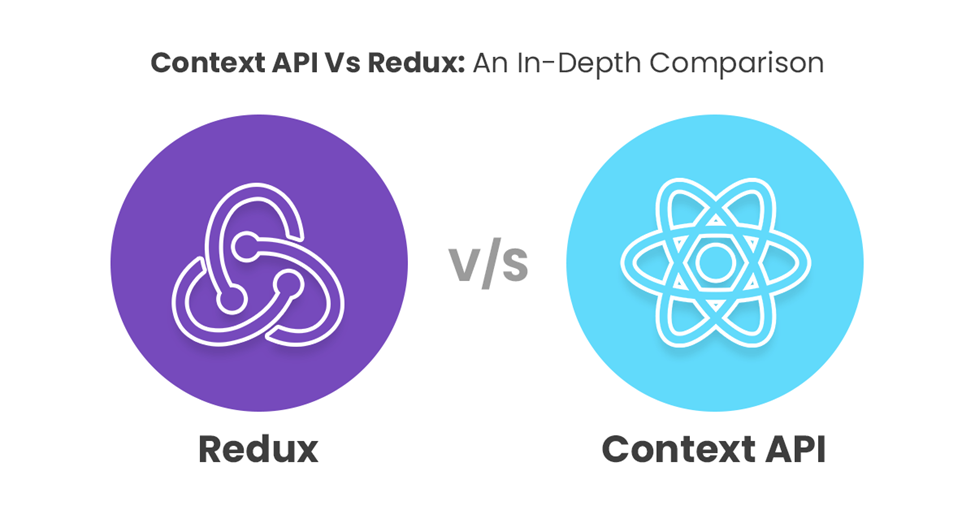
In MERN (MongoDB, Express, React, Node.js) applications, managing state effectively is critical, and both Redux and Context API are popular choices for state management. Each has its unique set of advantages that make it suitable for different types of applications.
Advantages of Using Redux in MERN Applications
- Centralized State Management: Redux stores the entire application’s state in a single global store, making it easier to manage and track the state across various components. This centralization simplifies debugging and tracing changes in complex applications.
- Predictable State Flow: Redux uses actions and reducers, which ensures that state changes happen in a predictable and structured manner. This makes the app more maintainable and reduces the chances of unintended side effects, as state changes only occur through dispatched actions.
- Middleware Support: Redux supports middleware such as Redux Thunk or Redux Saga, which are extremely helpful for managing asynchronous operations (like API calls). These middlewares allow actions to be dispatched asynchronously, which is crucial for data fetching in MERN applications.
- DevTools Integration: Redux comes with excellent development tools, such as the Redux DevTools Extension. These tools help developers inspect actions, state, and even time travel through previous states, aiding in debugging and testing.
- Scalability: Redux is ideal for large-scale applications with complex state logic. It helps prevent issues like prop drilling by allowing any component to access the global store directly. It’s well-suited for applications with multiple components that need access to a shared state.
Advantages of Using Context API in MERN Applications
- Simplicity: The Context API is built directly into React, which means it doesn’t require additional libraries or setup. It’s easier to integrate into small to medium-sized applications, where the complexity of managing state doesn’t warrant the overhead of Redux.
- Reduced Boilerplate: Unlike Redux, which requires actions, reducers, and store configurations, the Context API is more straightforward. You create a context, provide the state to the component tree, and consume it within any child component. This simplicity makes it quicker to implement for smaller projects.
- Less Overhead for Small Apps: In smaller applications where the state management needs are minimal, using the Context API can be more efficient than Redux. It prevents unnecessary complexity and makes the codebase more readable and easier to manage. Check the MERN Stack Certification courses to learn more.
- No Need for Third-Party Libraries: Since the Context API is built into React, you don’t need to rely on third-party libraries. This reduces the risk of dependency conflicts and makes your application lighter and faster to run.
- Better for Localized State: The Context API works well for passing data through the component tree without prop drilling. It’s particularly useful when you only need to share state among a few components or within a smaller scope, such as theme toggling or user authentication status.
Redux is suited for larger, more complex MERN applications that require robust state management, middleware support, and debugging tools. It excels in scalable applications that need a centralized state and a predictable flow of actions. Context API, on the other hand, is ideal for smaller applications or scenarios where the state management is simpler. It is easier to implement, with less boilerplate, and is perfect for applications with limited or localized state-sharing needs. Choosing between Redux and Context API depends on the application’s complexity and state management requirements. Consider checking the Next Js Course or MERN Stack courses for the best guidance.
Conclusion
In summary, Redux is best suited for large, complex MERN applications requiring centralized state management, scalability, and advanced debugging tools. Context API is more suitable for smaller projects with simpler state management needs, offering ease of use and minimal setup. The choice depends on the application’s scale and complexity.